순열(Permutation)
서로 다른 n개 중에서 r개를 취하여 그들을 일렬로 세울 때, 하나하나를 n개 중에서 r개 취한 순열
(= 서로 다른 n 개 중 r 개를 골라 순서를 고려해 나열한 경우의 수)
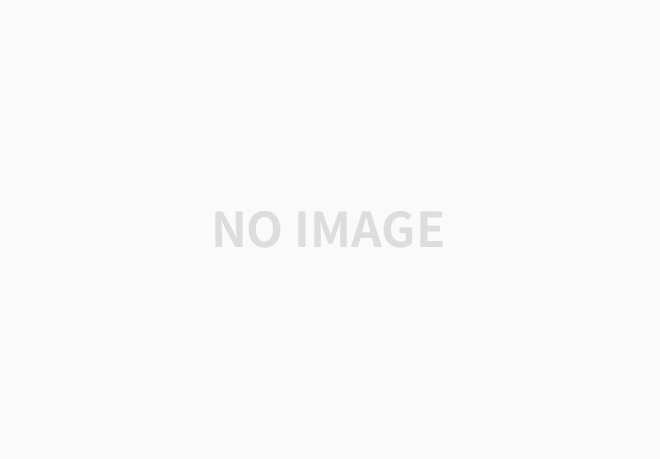
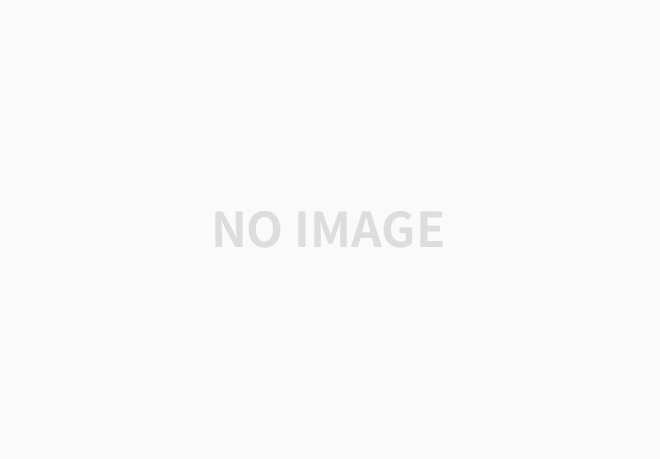
# 파이썬에서 순열을 사용하는 법
>>> from itertools import permutations
>>> test = [1,2,3,4,5]
>>> permutations(test)
<itertools.permutations object at 0x031FD988>
>>> list(permutations(test, 2))
[(1, 2), (1, 3), (1, 4), (1, 5), (2, 1), (2, 3), (2, 4), (2, 5), (3, 1), (3, 2),
(3, 4), (3, 5), (4, 1), (4, 2), (4, 3), (4, 5), (5, 1), (5, 2), (5, 3), (5, 4)]
조합(Combination)
서로 다른 n개 중에서 r개(n>=r)취하여 조를 만들 때, 이 하나하나의 조를 n개 중에서 r개 취한 조합
-> 순서가 필요하지 않다.
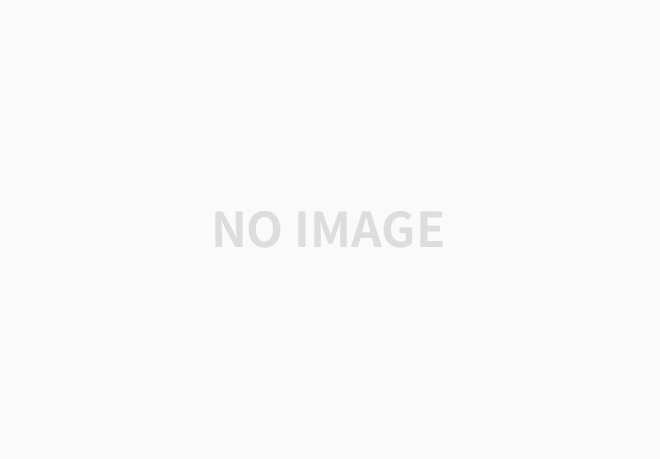
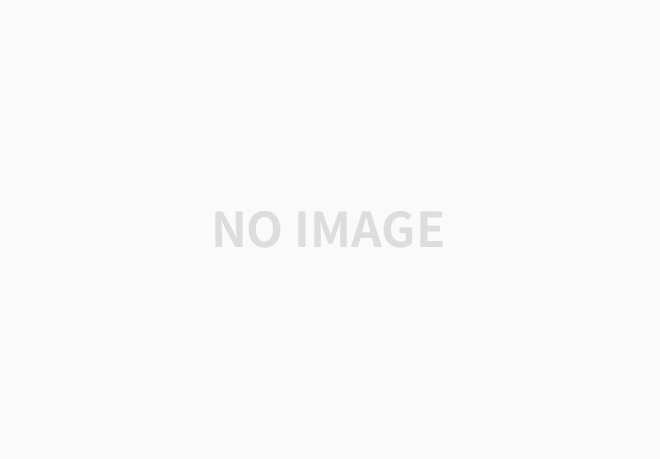
# 파이썬에서 조합을 사용하는 법
>>> from itertools import combinations
>>> test = [1,2,3,4,5]
>>> list(combinations(test,2))
[(1, 2), (1, 3), (1, 4), (1, 5), (2, 3), (2, 4), (2, 5), (3, 4), (3, 5), (4, 5)]
>>> test = [1,1,2,3,4,5]
>>> list(combinations(test,2))
[(1, 1), (1, 2), (1, 3), (1, 4), (1, 5), (1, 2), (1, 3), (1, 4),
(1, 5), (2, 3), (2, 4), (2, 5), (3, 4), (3, 5), (4, 5)]
Product
데카르트 곱(cartesian product)
집합 A의 원소 a와 집합 B의 원소 b를 순서대로 벌여 놓고 괄호로 묶은 순서쌍 (a,b) 전체의 집합
# 파이썬에서 product 사용하는 법
>>> from itertools import product
>>> test = [1,2,3,4,5]
>>> list(product(test,repeat = 2))
[(1, 1), (1, 2), (1, 3), (1, 4), (1, 5), (2, 1), (2, 2), (2, 3), (2, 4), (2, 5),
(3, 1), (3, 2), (3, 3), (3, 4), (3, 5), (4, 1), (4, 2), (4, 3), (4, 4), (4, 5),
(5, 1), (5, 2), (5, 3), (5, 4), (5, 5)]
* 두 개 이상의 리스트의 모든 조합 Product 사용하여 구하기
- 리스트 2개인 경우
# 리스트 두 개
>>> from itertools import product
>>> t1 = [1, 2, 3, 4, 5]
>>> t2 = ['A', 'B', 'C', 'D']
>>> list(product(t1, t2))
[(1, 'A'), (1, 'B'), (1, 'C'), (1, 'D'), (2, 'A'), (2, 'B'), (2, 'C'), (2, 'D'), (3, 'A'), (3, 'B'),
(3, 'C'), (3, 'D'), (4, 'A'), (4, 'B'), (4, 'C'), (4, 'D'), (5, 'A'), (5, 'B'), (5, 'C'), (5, 'D')]
- 리스트 3개인 경우
# 리스트 세 개
>>> from itertools import product
>>> t1 = [1, 2, 3, 4, 5]
>>> t2 = ['A', 'B', 'C', 'D']
>>> t3 = ['가', '나']
>>> list(product(t1, t2, t3))
[(1, 'A', '가'), (1, 'A', '나'), (1, 'B', '가'), (1, 'B', '나'), (1, 'C', '가'), (1, 'C', '나'), (1, 'D', '가'),
(1, 'D', '나'), (2, 'A', '가'), (2, 'A', '나'), (2, 'B', '가'), (2, 'B', '나'), (2, 'C', '가'), (2, 'C', '나'),
(2, 'D', '가'), (2, 'D', '나'), (3, 'A', '가'), (3, 'A', '나'), (3, 'B', '가'), (3, 'B', '나'), (3, 'C', '가'),
(3, 'C', '나'), (3, 'D', '가'), (3, 'D', '나'), (4, 'A', '가'), (4, 'A', '나'), (4, 'B', '가'), (4, 'B', '나'),
(4, 'C', '가'), (4, 'C', '나'), (4, 'D', '가'), (4, 'D', '나'), (5, 'A', '가'), (5, 'A', '나'), (5, 'B', '가'),
(5, 'B', '나'), (5, 'C', '가'), (5, 'C', '나'), (5, 'D', '가'), (5, 'D', '나')]
- 이차원 리스트
# 이차원 리스트
>>> from itertools import product
>>> t = [[1, 2, 3], ['가', '나'] ,['A', 'B']]
>>> list(product(*t))
[(1, '가', 'A'), (1, '가', 'B'), (1, '나', 'A'), (1, '나', 'B'), (2, '가', 'A'), (2, '가', 'B'),
(2, '나', 'A'), (2, '나', 'B'), (3, '가', 'A'), (3, '가', 'B'), (3, '나', 'A'), (3, '나', 'B')]
- TypeError: object of type 'itertools.combinations' has no len() 오류
조합을 사용한 후 바로 len() 함수를 사용하면 error발생함 list로 형변환한 뒤 len()을 사용해야함
# error 발생
>>> a = combinations(test,2)
>>> a
<itertools.combinations object at 0x031FD910>
>>> len(a)
Traceback (most recent call last):
File "<pyshell#13>", line 1, in <module>
len(a)
TypeError: object of type 'itertools.combinations' has no len()
#list로 형변환
>>> a = list(combinations(test,2))
>>> a
[(1, 1), (1, 2), (1, 3), (1, 4), (1, 5), (1, 2), (1, 3), (1, 4), (1, 5), (2, 3), (2, 4), (2, 5), (3, 4), (3, 5), (4, 5)]
>>> len(a)
15
product, permutations, combinations 차이
>>> from itertools import product, permutations, combinations
>>> test = ['A','B']
# product 데카르트의 곱
>>> list(product(test, repeat=2))
[('A', 'A'), ('A', 'B'), ('B', 'A'), ('B', 'B')]
# permutations 순열 - 순서 고려해 나열
>>> list(permutations(test,2))
[('A', 'B'), ('B', 'A')]
# combinations 조합 - 순서 고려하지 않음
>>> list(combinations(test,2))
[('A', 'B')]
'Study > Python study' 카테고리의 다른 글
[Python(파이썬)] 올림, 내림, 반올림 (소수점, 일의 자리, 십의 자리, ...) (0) | 2021.07.07 |
---|---|
[Python(파이썬)] 리스트, 튜플, 세트, 딕셔너리 (0) | 2021.07.06 |
[Python(파이썬)] 몫, 나머지 구하기( /, //, %, divmod() ) (0) | 2021.07.02 |
[Python(파이썬)] 문자열 인덱스로 접근하기 (0) | 2021.07.02 |
[Python(파이썬)] Numpy 라이브러리 (0) | 2021.07.01 |
댓글